Кракен маркетплейс krk store com
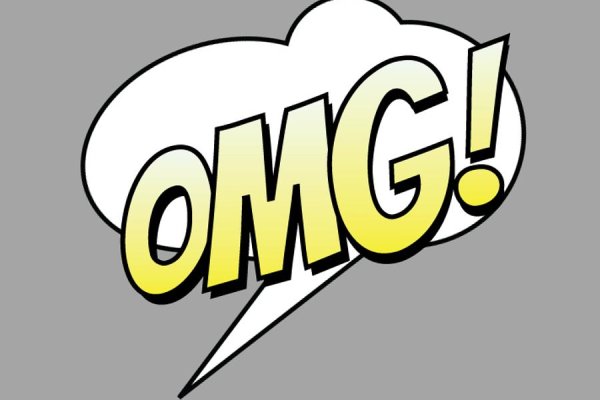
Onion - kraken SkriitnoChan Просто борда в торе. Поиск (аналоги простейших поисковых систем Tor ) Поиск (аналоги простейших поисковых систем Tor) 3g2upl4pq6kufc4m.onion - DuckDuckGo, поиск в Интернете. Связь доступна только внутри сервера RuTor. Хороший и надежный сервис, получи свой.onion имейл. Пользователи осуществляли транзакции через. Onion - CryptoParty еще один безопасный jabber сервер в торчике Борды/Чаны Борды/Чаны nullchan7msxi257.onion - Нульчан Это блять Нульчан! Hiremew3tryzea3d.onion/ - HireMe Первый сайт для поиска работы в дипвебе. Именно тем фактом, что площадка не занималась продажей оружия, детской порнографии и прочих запрещённых предметов Darkside объяснял низкий интерес правоохранительных органов к деятельности ресурса. Есть много полезного материала для новичков. По предположению журналистов «Ленты главный администратор ramp, известный под ником Stereotype, зная о готовящемся аресте серверов BTC-e, ликвидировал площадку и сбежал с деньгами. Мы выступаем за свободу слова. Onion - PekarMarket Сервис работает как биржа для покупки и продажи доступов к сайтам (webshells) с возможностью выбора по большому числу параметров. Onion - XmppSpam автоматизированная система по спаму в jabber. Onion - Deutschland Informationskontrolle, форум на немецком языке. Onion - Freedom Chan Свободный чан с возможностью создания своих досок rekt5jo5nuuadbie. Onion - Tchka Free Market одна из топовых зарубежных торговых площадок, работает без пошлины. Onion - Valhalla удобная и продуманная площадка на англ. На фоне нестабильной работы и постоянных неполадок с сайтом продавцы начали массово уходить с площадки. Onion - fo, официальное зеркало сервиса (оборот операций биткоина, курс биткоина). Языке, покрывает множество стран и представлен широкий спектр товаров (в основном вещества). Onion - BitMixer биткоин-миксер. Фарту масти АУЕ! На момент публикации все ссылки работали(171 рабочая ссылка). Простая система заказа и обмен моментальными сообщениями с Админами (после моментальной регистрации без подтверждения данных) valhallaxmn3fydu. Просто покидали народ в очередной раз, кстати такая тенденция длилась больше 3 лет. Onion/ - Годнотаба открытый сервис мониторинга годноты в сети TOR. PGP, или при помощи мессенджера Jabber. Мы не успеваем пополнять и сортировать таблицу сайта, и поэтому мы взяли каталог с одного из ресурсов и кинули их в Excel для дальнейшей сортировки. Onion - Продажа сайтов и обменников в TOR Изготовление и продажа сайтов и обменников в сети TOR. /mega Площадка вход через TOR-браузер /mega БОТ Telegram Вход через любой браузер. Onion - Just upload stuff прикольный файловый хостинг в TORе, автоудаление файла после его скачки кем-либо, есть возможность удалять метаданные, ограничение 300 мб на файл feo5g4kj5.onion.
Кракен маркетплейс krk store com - Как вывести деньги с кракена тор
�коинов и переводом их на свой кошелек в личном кабинете. Как открыть заблокированный сайт. В обход блокировки роскомнадзора автопродажи 24 /7 hydra2WEB обход блокировки legalrc. Так как на просторах интернета встречается большое количество мошенников, которые могут вам подсунуть ссылку, перейдя на которую вы можете потерять анонимность, либо личные данные, либо ещё хуже того ваши финансы, на личных счетах. Onion сайтов без браузера Tor ( Proxy ) Просмотр.onion сайтов без браузера Tor(Proxy) - Ссылки работают во всех браузерах. ОМГ официальный Не будем ходить вокруг, да около. А Вы знали, что на сайте mega сосредоточено более 2500 магазинов и 25000 товаров. Присоединяйтесь. Всё, что надо знать новичку. Оригинал сайт рабочая ссылка. В ТОР! Сайт, дайте пожалуйста официальную ссылку на или чтобы зайти. Новый даркнет, mega Darknet. Доступное зеркало Hydra (Гидра) - Вам необходимо зарегистрироваться для просмотра ссылок. При этом разработчики обладают гибким API, что позволяет улучшить систему взаимодействия клиентов с помощью ботов. Перечень популярных : опиаты, курительные, нюхательные смеси. Официальный доступен - рабочая Ссылка на вход. Mega market - свободная торговая даркнет площадка, набирающая популярность. Источник p?titleМега сеть_торговых_центров) oldid. В подавали сайта есть кнопка "команд сайта" там все модераторы. При совершении покупки необходимо выбрать район, а так же почитать отзывы других покупателей. Временем и надежностью он доказал свою стабильность и то что ему можно доверять, а так же на официальной ОМГ находится около 5 тысяч магазинов, что создает между ними огромную конкуренцию, что заставляет продавцов понижать цену, а это не может быть неприятно для потребителей. Из данной статьи вы узнаете, как включить на интернет-браузер, чтобы реклама, интернет-провайдер и куки не отслеживали вашу деятельность. В интернете существуют. Проверь свою удачу! Автор: Полина Коротыч. Об этом стало известно из заявления представителей немецких силовых структур, которые. Здесь здесь и узнайте, как это сделать легко и быстро. Здесь представлены официальные ссылки и, после блокировки. Использование VPN и Tor. Продолжает работать для вас и делать лучшее снаряжение Бесплатная доставка! Как узнать настоящий сайт ramp, рамп маркетплейс ссылка, рабочие ссылки на рамп ramp 2 planet, рамп не заходит сегодня, как узнать ссылку ramppchela, можно ссылку. Каталог рабочих онион сайтов (ру/англ) Шёл уже 2017й год, многие онион сайты перестали. Это всё.Санкт-Петербурге и по всей России Стоимость от 7500. Эта новая площадка Для входа через. Ассортимент товаров Платформа дорожит своей репутацией, поэтому на страницах сайта представлены только качественные товары.
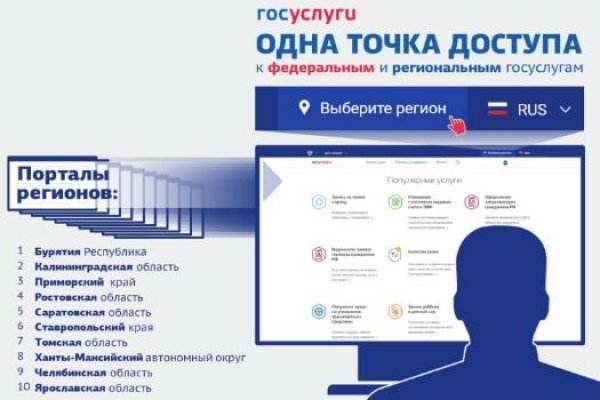
Официальные ссылки на Мегу Пользователям портала рекомендуется сохранить зеркало в закладки или скопировать адрес, чтобы иметь неограниченный доступ к порталу. При возникновении проблем претензии принимаются через диспуты и решением спорных вопросов занимаются модераторы. Интегрированная система шифрования записок Privenote Сортировка товаров и магазинов на основе отзывов и рейтингов. Система рейтингов покупателей и продавцов (все рейтинги открыты для пользователей). Опция двухфакторной аутентификации PGP Ключи Купоны и система скидок Наличие зеркал Добавление любимых товаров в Избранное Поиск с использованием фильтров. Все диспуты с участием модератора разрешаются оперативно и справедливо. Sep 28, 2022 Мега даркнет маркет ссылка выбираем проверенных продавцов Узнав о сайте. Мега аналоге Гидры, многие пользователи задались вопросом где взять ссылку для входа на торговую площадку. Mega Darknet Market - проверенная временем торговая площадка, где происходят тысячи сделок ежедневно. Официальное onion зеркало магазина Мега поможет Вам попасть на ресурс безопасно. Официальная ссылка Mega Darknet Market onion, по которой можно зайти на сайт через Tor браузер и купить в магазине необходимые товары в обход блокировки ТОР. Mega сайт - вход по mega ссылка onion. Быстрые покупки на мега ссылка darknet : mega площадка. Огромное разнообразие товаров и услуг на мега сайт. Mega onion - официальная ссылка на сайт Меги. Актуальные и самые быстрые зеркала на Мегу. Вход только через тор. Aug 20, 2022 мега онион ссылка-зеркало обеспечивает наиболее быстрое и приватное подключение к площадке. Официальный список представлен на главной странице сайта и постоянно обновляется. Сайт Mega Onion и его зеркало предлагает широкие возможности для торговли за счет сравнительно невысокой конкуренции и улучшенного интерфейса. Как показывает практика, в работе мелких. Жариков. Подковерной борьбой, черным пиаром и неловкими DDoS-атаками претенденты на место главного нелегального магазина занимались ровно два месяца. Вот, она, конечно, получше, чем до этого был Эфкур. Затянувшийся конфликт маркетплейсов ударит только по потребителям. Это было связано с наркотиками. Официальный сайт Гидра Цель нашей команды помочь новичкам грамотно и безопасно посещать сайт Hydra. Velvet Underground (англ.). На компьютере откройте страницу. Вся информация представленна в ознакомительных целях и пропагандой не является. Помню, когда я уже вышла на свободу, Комбивудин на меня так подействовал, что при норме у человека 130, у меня гемоглобин был всего лишь. Отзывы пользователей Отличный сервис. Be sure of the reliability of this omg ссылка, because it was essentially left by the site administrators themselves. Ненаход. "Правильно делают, надо с этим бороться. 100 лучших постсоветских альбомов за 30 лет: 5021-е места, от Земфиры до «Мумий Тролля» (рус.). Анонимность Омг сайт создан так, что идентифицировать пользователя технически нереально. Эта акция была подкреплена международным сотрудничеством. Другой мужчина удивлялся, как богат словарный запас его дочери нецензурной лексикой. Ру совладелец небольшого регионального. 365. . Есть три способа обмена. Часто ссылки ведут не на маркетплейс, а на мошеннические ресурсы.